Webpack和NPM优化前端应用的教程
2024-10-18
微博文章:高效应用构建之路——Webpack、NPM与树摇优化
摘要
在Web开发的世界中,性能优化是至关重要的。一种有效的方法是使用“树摇”和压缩技术,这两者可以帮助优化代码并提高应用程序的加载速度及整体效率。
本文将探索如何通过Webpack和npm集成来实现这些优化——以一个虚构项目的开发为例。
什么是“树摇”与“压缩”
树摇:这是指对大型JavaScript文件中的某些部分进行分析,去除那些从未使用的代码,从而产生更小的代码。这个过程由优化器(通常为Webpack)执行,通过源地图来确定哪些函数或变量从未被访问。
压缩:压缩是减少JavaScript代码大小的过程,通过删除不必要的空白、注释和其他不影响功能的字符来实现。
案例背景
假设我们有一个电子商务网站,前端组件用于显示产品列表。我们的前端应用将使用React进行渲染,并在Node.js后端部署。让我们看看如何使用Webpack和npm这两种技术来达到这些优化的目的。
设置项目环境
-
安装Webpack与NPM:
npm install --save-dev webpack webpack-cli
-
创建
webpack.config.js
文件:const path = require('path'); module.exports = { entry: './src/index.ts', output: { filename: 'bundle.js' }, resolve: { extensions: ['.tsx', '.ts', '.js'] } };
-
将TypeScript编译为JavaScript:
tsc --module esnext
-
创建一个名为
src
的文件夹并编写必要的文件:-
创建一个
index.ts
文件,这是开发应用时的第一部分代码:import React from 'react'; import './styles.css'; const App = () => { return <h1>Hello, World!</h1>; }; export default App;
-
开发流程
假设我们正在构建一个电子商务网站,在这个过程中涉及不同的模块和组件。每个模块完成后,需要使用Webpack将其编译并打包。
步骤1:开发App.tsx
import React from 'react';
import './styles.css';
const App = () => {
return <h1>Hello, World!</h1>;
};
export default App;
运行Webpack进行编译与打包
我们可以通过命令行来运行Webpack:
npx webpack --mode development
在运行了这个命令后,将在./dist/
目录中生成一个名为bundle.js
的文件。
使用NPM包管理器
然后,让我们看看如何使用npm进行项目模块的导入和打包。假设我们有一个小脚本用于发送HTTP请求:
import axios from 'axios';
我们可以像处理其他任何模块一样去包裹它来引用npm包:
npx webpack --mode production
树摇示例
在App.tsx
组件中,应该使用“树摇”来避免不必要的代码被编译并打包:
import React from 'react';
import './styles.css';
const App = () => {
return <h1>Hello, World!</h1>;
};
export default App;
Webpack会分析这个文件中的tsconfig.json
(或.js
的ES模块版本)配置,并去除不必要的代码:
npx webpack --mode production
压缩示例
为了进一步优化我们的应用,我们可以在我们的webpack配置文件中添加“optimization”选项:
const path = require('path');
module.exports = {
entry: './src/index.ts',
output: {
filename: 'bundle.js'
},
resolve: {
extensions: ['.tsx', '.ts', '.js']
},
optimization: true,
};
然后运行Webpack,我们可以使用以下命令进行压缩:
npx webpack --mode production --config webpack.config.js
总结
通过理解树摇和压缩工作原理,我们可以在前端应用中显著优化性能。这种方法不仅可以改善开发过程,还能提供一种可靠的环境来部署和维护现代的Web应用程序。
使用Webpack和npm不仅提高了开发效率,还提供了强大的构建系统,并在生产环境中可以有效地进行维护。 Certainly! Below is the information presented in a tabular format:
Component | Functionality |
---|---|
Webpack & npm | Integrated to enhance application performance through optimization and package management. |
Tree Shaking | Eliminates unused code, reducing file size and improving overall app speed. |
Compression | Reduces the size of JavaScript files by eliminating unnecessary characters (blank spaces, comments, etc.). |
Application Flow | A fictional scenario where a shopping website is developed with React for front-end rendering and Node.js for backend. |
Development Phase |
- Installation:
npm install --save-dev webpack webpack-cli
- Configuration (
webpack.config.js
):const path = require('path'); module.exports = { entry: './src/index.ts', output: { filename: 'bundle.js' }, resolve: { extensions: ['.tsx', '.ts', '.js'] } };
- TypeScript Compilation:
tsc --module esnext
- Source Map for Debugging (
./dist/bundle.js.map
) -
index.ts
file for the first piece of code in React development. - Node.js Module Import:
import axios from 'axios';
| Running Webpack | By using npx webpack --mode development
, you can compile and bundle your TypeScript or JavaScript files as needed.
By running npx webpack --mode production
, you can also create optimized builds for production use. |
| Production Build | A production build of the application would be achieved by using npx webpack --mode production
which compiles all modules in the project into optimized bundles suitable for deployment or further optimization.
For example, running npx webpack --config webpack.config.js --mode production
will create and optimize an output bundle named bundle.js
. |
| Tree Shaking Example | In the App.tsx
file (assuming a React application), removing unused code from .tsx
or .ts
files results in smaller bundles. Using webpack --mode production
ensures only necessary parts are included, thus reducing weight and improving performance.
| Compression Example | Implementing optimization in webpack can lead to "tree shaking" where unused portions of code are eliminated, enhancing application speed by diminishing the size of JavaScript files. |
| Optimization Settings | Setting optimization: true
in webpack's configuration file enables various optimization features like tree shaking and dead-code elimination that help reduce both size and runtime overhead. |
This tabular view provides a clear comparison between Webpack, npm, and their integration capabilities for performance optimization in JavaScript-based projects.
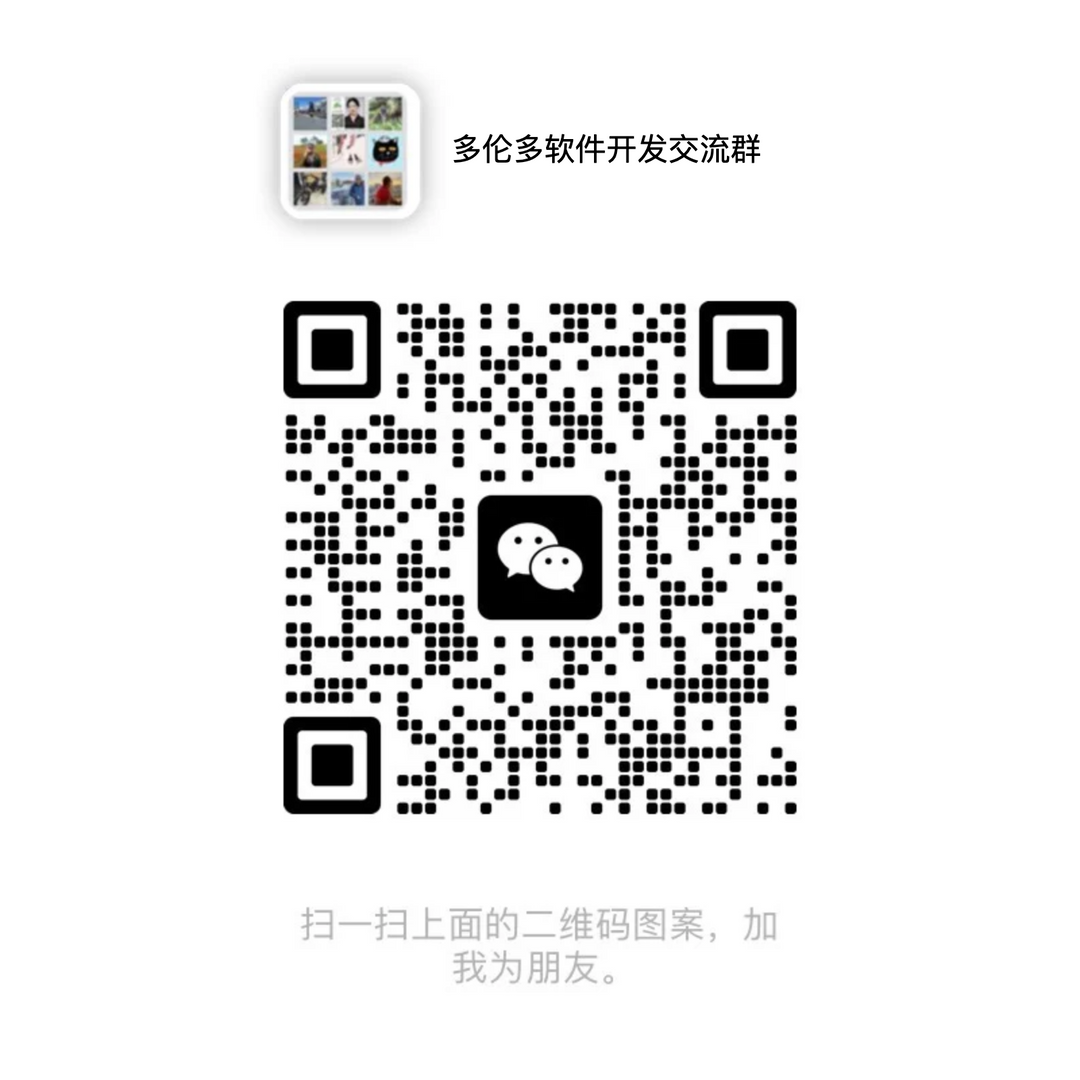