使用Code Splitting提高Webpack性能
2024-10-18
示例场景:复杂的单页面应用程序
想象一下,你的网站包含几个不同的页面,每个页面都需要特定的特性。例如,“关于我们”,“产品”和“联系”。这些页面需要不同的功能,比如处理用户交互或动态渲染部分页面。
目前,你正在使用 Webpack
来捆绑所有这些脚本文件和其他依赖项在名为 main.js
的单一文件中。这种做法对于小型项目是有效的,但是当处理大型和复杂的项目时会变得困难。
问题:
当你加载多个模块时,在 JavaScript 引擎(如 V8)中执行时遇到了性能问题。引擎必须等待每个模块都完全加载完毕后才能执行其代码。这会导致由于需要等待所有内容加载完成而产生的额外开销,从而影响性能。
解决方案:Code Splitting
Code splitting
是一种技术,它有助于提高应用程序的性能,并通过 Webpack 的功能 splitChunks
来实现。
步骤:
-
识别模块:首先,确定你想要在特定时刻加载哪些脚本或捆绑文件。
-
使用 Webpack 配置中的
entryPoints
:// webpack.config.js const path = require('path'); module.exports = { entry: { main: ['react', 'bootstrap'], secondSection: './src/second-section', thirdSection: './src/third-section' }, output: { filename: '[name].js' }, optimization: { splitChunks: { cacheGroups: { vendor: { test: /[\\/]node_modules[\\/]/, name: 'vendors', chunks: 'all' } } } } };
-
更新
main.js
以使用代码分割:// main.js import React from 'react'; import bootstrap from './bootstrap'; function App() { return <div className="App">Hello, World!</div>; } export default App;
-
为每个部分的单独捆绑文件动态导入:
每个部分的捆绑文件可以根据不同的路由动态加载。
// second-section.js import React from 'react'; import ReactDOM from 'react-dom'; function SecondSection() { return ( <div> <h1>第二部分</h1> {dynamicImports()} </div> ); } const dynamicImports = async () => { try { await Promise.all([ import('./second-section-components'), // 添加更多导入项以加载其他功能 ]); console.log('组件已加载'); } catch (error) { console.error('错误加载组件:', error); } }; export default SecondSection;
-
在主应用中使用
dynamicImports
:// main.js import React from 'react'; import bootstrap from './bootstrap'; function App() { return <div className="App">Hello, World!</div>; } export default App; const dynamicImports = async () => { try { await Promise.all([ import('./second-section'), // 添加更多导入项以加载其他功能 ]); console.log('组件已加载'); } catch (error) { console.error('错误加载组件:', error); } }; export default dynamicImports;
结论:
通过使用 Code splitting
,结合 Webpack 的功能 splitChunks
,你可以显著提高单页面应用程序的性能。而不是等待所有内容都完全加载后才开始执行,你可以在任何时刻只加载你需要的内容,从而减少了初始化加载时间并改善了用户体验。
下一步:
对于大型应用程序,考虑其他优化技术也非常有帮助,例如使用 Webpack 的 Hot Module Replacement (HMR)
和 Browserify
或 Rollup
来动态捆绑脚本。这将让你更有效地管理你的应用程序的依赖项,甚至进一步优化性能。 Sure! Below is an example of how to implement Code Splitting and use Webpack's splitChunks
feature to optimize your single page application (SPA).
Step 1: Define Entry Points
Define the entry points where Webpack will bundle different components. For instance, you might have a main app entry point that includes React and Bootstrap:
// webpack.config.js
const path = require('path');
module.exports = {
entry: {
main: ['react', 'bootstrap'],
secondSection: './src/second-section',
thirdSection: './src/third-section'
},
output: {
filename: '[name].js'
},
optimization: {
splitChunks: {
cacheGroups: {
vendor: {
test: /[\\/]node_modules[\\/]/,
name: 'vendors',
chunks: 'all'
}
}
}
}
};
Step 2: Update main.js
to Use Code Splitting
The main entry point will now use dynamic imports for each section it needs:
// main.js
import React from 'react';
import bootstrap from './bootstrap';
function App() {
return <div className="App">Hello, World!</div>;
}
export default App;
Step 3: Update Section Bundles
For each section (e.g., secondSection
, thirdSection
), define the imports and create a function that loads them dynamically:
// second-section.js
import React from 'react';
import ReactDOM from 'react-dom';
function SecondSection() {
return (
<div>
<h1>第二部分</h1>
{dynamicImports()}
</div>
);
}
const dynamicImports = async () => {
try {
await Promise.all([
import('./second-section-components'),
// Add more imports to load other features
]);
console.log('组件已加载');
} catch (error) {
console.error('错误加载组件:', error);
}
};
export default SecondSection;
Step 4: Use dynamicImports
in the Main Application
The main application will use a dynamic function to load all necessary components:
// main.js
import React from 'react';
import bootstrap from './bootstrap';
function App() {
return <div className="App">Hello, World!</div>;
}
export default App;
const dynamicImports = async () => {
try {
await Promise.all([
import('./second-section'),
// Add more imports to load other features
]);
console.log('组件已加载');
} catch (error) {
console.error('错误加载组件:', error);
}
};
export default dynamicImports;
Conclusion:
By using Webpack's splitChunks
feature and implementing Code Splitting, you can significantly improve the performance of your SPA. Rather than waiting for all content to load, you only bundle and load what is necessary at each step, which reduces the initial loading time and enhances user experience.
For even better optimization, consider other techniques such as using Webpack's Hot Module Replacement (HMR) and Browserify
or Rollup
to dynamically bundle scripts. This will help manage dependencies more effectively and further improve performance.
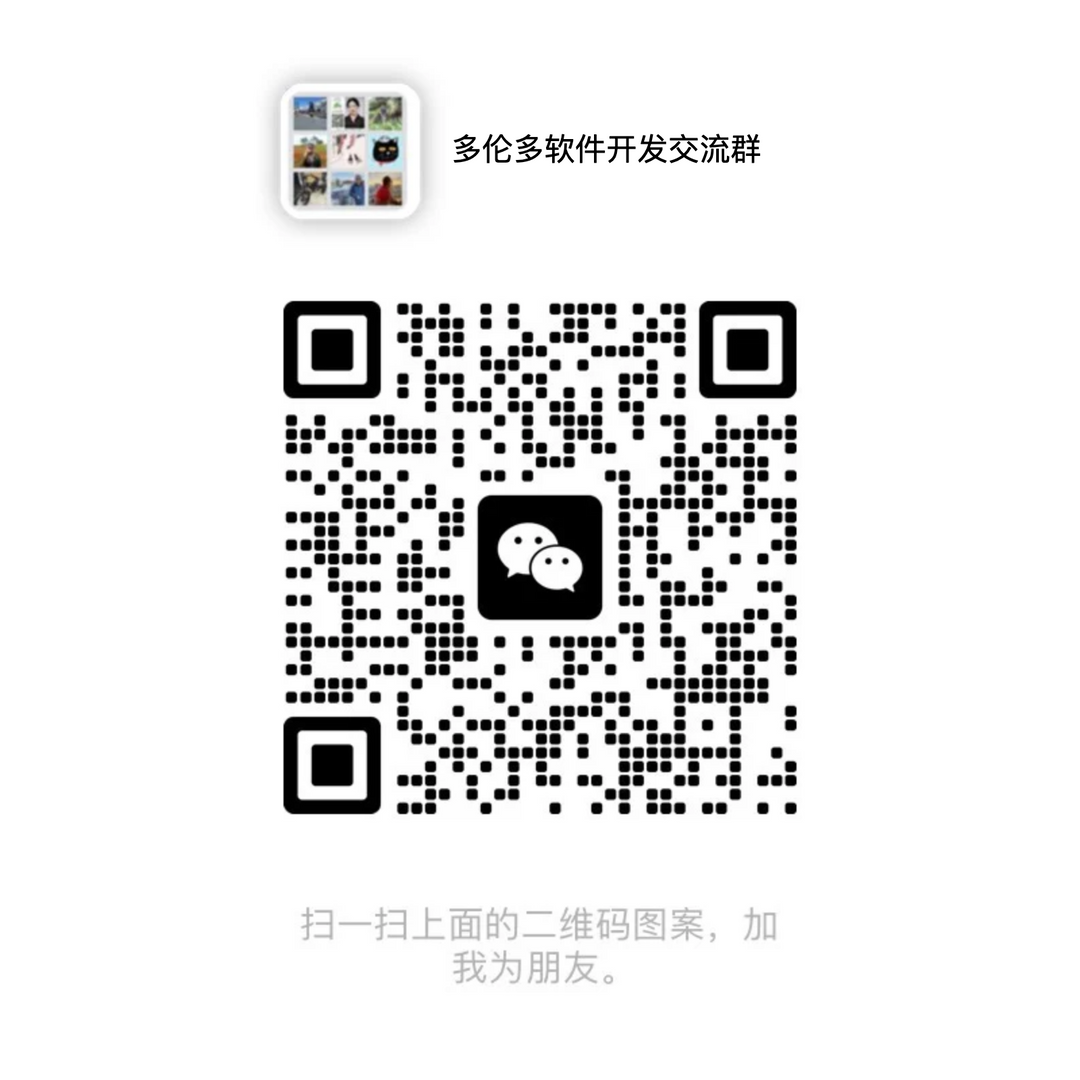