使用 `XMLHttpRequest` 实现异步请求与即时反馈
2024-10-18
异步编程的力量:在前端开发中的 XMLHttpRequest
对象
今天数字世界中快速发展的步伐,前端开发者正在寻找提高用户体验和提升应用程序性能的方法。一种成为越来越流行的技术就是使用 JavaScript 的 XMLHttpRequest
对象进行异步编程。这篇文章将深入探讨如何利用 XMLHttpRequest
这种方法来处理请求的异步性,从而使网络开发过程更加高效。
什么是 XMLHttpRequest
XMLHttpRequest
对象允许在 Web 页面与服务器之间实现无阻塞通信,而无需用户重新加载整个页面。它是浏览器在网络栈上低层次接口的一种形式,为从 JavaScript 运行在浏览器中的代码中方便地发送 HTTP 请求提供了一种方式。
实际应用场景
让我们来看一个场景,在构建一个带有评论功能的博客应用时。这个应用允许用户提交文章评论,并且这些评论需要存储在单独运行 PHP 脚本的页面上。因为处理这些复杂度较高的服务器端逻辑可能会花费一些时间,所以这个问题就出现了:当用户提交评论时,前端需要立即获得反馈,比如评论是否被添加了。
问题陈述
- 当用户提交一个评论时,前端需要即刻反馈,比如评论是否被添加了。
- 用户期望他们的评论在提交后会立刻显示出来。
使用 XMLHttpRequest
解决方案
-
用户提交评论:
前端代码检查表单提交是否有错误,并然后使用
XMLHttpRequest
发送一个 POST 请求到 PHP 脚本:
function submitComment() {
var xhr = new XMLHttpRequest();
var url = "processComment.php"; // 这是处理评论的 PHP 脚本的 URL
xhr.open('POST', url, true);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.onreadystatechange = function () {
if (xhr.readyState == 4 && xhr.status == 200) {
document.getElementById('commentForm').reset(); // 重置表单以防止重复提交
showComment(xhr.responseText); // 在页面上显示评论
}
};
xhr.send("comment=" + encodeURIComponent(document.querySelector('#new-comment-input').value));
}
- 处理评论的 PHP 脚本: 这个 PHP 脚本处理表单提交,并生成一个 XML 文件。然后通过 AJAX 返回给前端。
<?php
// 处理表单提交并使用你的服务器端逻辑
$xml = simplexml_load_file('comments.xml'); // 假设有一个名为 comments.xml 的文件
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$comment = $_POST['comment'];
$xml->addChild('Comment', $comment); // 在 XML 中添加评论
file_put_contents('comments.xml', (string)$xml);
echo json_encode(['success' => true, 'message' => '评论已成功提交']);
}
?>
- 显示评论: 首先,当服务器返回一个成功信息时,在前端的表单上重置表单,并在页面上显示评论。
function showComment(response) {
var commentContainer = document.querySelector('#comments');
if (response.success === true) {
var newComment = document.createElement('div');
newComment.className = 'comment';
var commentText = response.message;
newComment.textContent = `Posted by: ${commentText}`;
commentContainer.appendChild(newComment);
} else {
alert(response.message); // 处理错误
}
}
总结
使用 XMLHttpRequest
能够使开发人员进行异步请求,提供即时反馈并优化用户交互。这种技术在前端开发中至关重要,因为它可以帮助你简化你的前端应用程序,确保页面加载更快,并提升交互体验。这是一种在当今快速发展的网络开发环境中的关键技能。
通过将 AJAX 结合 XMLHttpRequest
,你可以有效地加快你的前端应用的处理速度、减少页面重新加载的时间,从而提供更好的用户体验。 | 概述 | 无阻塞通信 | 异步编程 |
| --- | --- | --- |
| 含义 | 在 Web 页面与服务器之间实现无阻塞通信,而无需用户重新加载整个页面。 | 提供即刻反馈和处理复杂度较高的逻辑的解决方案。 |
| 使用场景 | 1. 用户提交评论时:立即显示评论状态。
2. 数据请求时:例如,查询用户的个人信息、新闻文章等数据。
3. 处理服务器端逻辑较复杂时。 | 1. 网络请求处理延迟带来的问题:用户提交的表单数据需要在服务器上进行处理。
2. 需要立即获取结果的异步任务如动画效果、加载状态指示器等。 |
| 技术栈 | JavaScript, XMLHttpRequest, AJAX (Asynchronous JavaScript and XML) | JavaScript, jQuery (for AJAX), Axios, Fetch API, etc. |
| 示例代码 |
- 前端提交评论:
function submitComment() {
var xhr = new XMLHttpRequest();
var url = "processComment.php"; // 这是处理评论的 PHP 脚本的 URL
xhr.open('POST', url, true);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.onreadystatechange = function () {
if (xhr.readyState == 4 && xhr.status == 200) {
document.getElementById('commentForm').reset(); // 重置表单以防止重复提交
showComment(xhr.responseText); // 在页面上显示评论
}
};
xhr.send("comment=" + encodeURIComponent(document.querySelector('#new-comment-input').value));
}
- 处理评论的 PHP 脚本:
<?php
// 处理表单提交并使用你的服务器端逻辑
$xml = simplexml_load_file('comments.xml'); // 假设有一个名为 comments.xml 的文件
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$comment = $_POST['comment'];
$xml->addChild('Comment', $comment); // 在 XML 中添加评论
file_put_contents('comments.xml', (string)$xml);
echo json_encode(['success' => true, 'message' => '评论已成功提交']);
}
?>
- 显示评论:
function showComment(response) {
var commentContainer = document.querySelector('#comments');
if (response.success === true) {
var newComment = document.createElement('div');
newComment.className = 'comment';
var commentText = response.message;
newComment.textContent = `Posted by: ${commentText}`;
commentContainer.appendChild(newComment);
} else {
alert(response.message); // 处理错误
}
}
总结 | 异步编程的工具和技术栈的选择可以根据需求和项目具体情况进行调整。 | 提供即时反馈和处理复杂度较高的逻辑的解决方案,适用于网络请求处理延迟及需要立即获取结果的情况。 |
---|
通过这些技术,你可以使前端应用更加高效、响应更快,并且更好地满足用户需求。
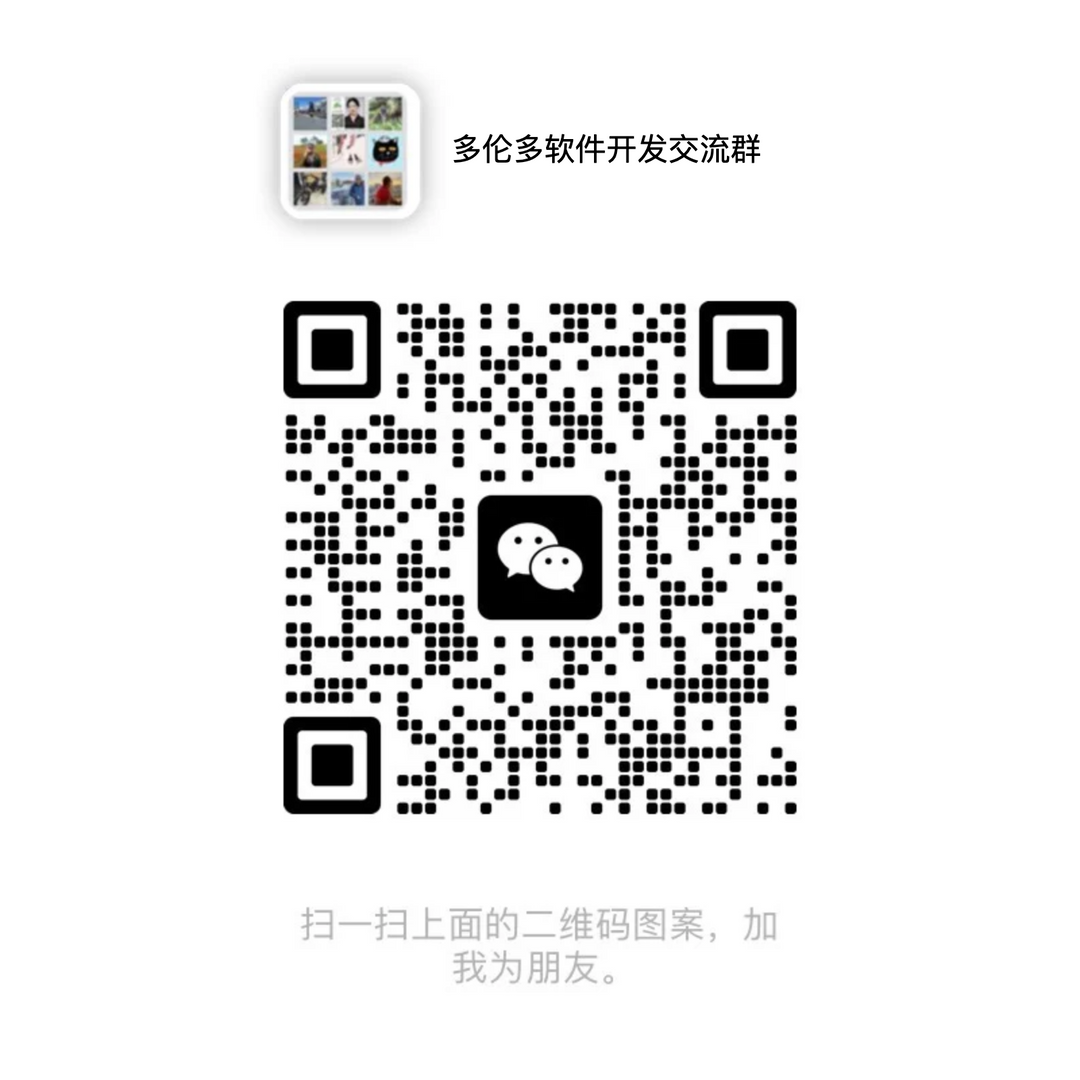