"Integrating Numerical Weather Prediction with Wind Field Representation and Parameterization for Coastal Forecasting"
2024-10-16
Title: The Intersection of Numerical Weather Prediction, Wind Field Representation, and Tide Modeling: A Case Study in Coastal Environments
Introduction
Coastal regions are dynamic systems that are heavily influenced by wind, water, and temperature fluctuations. Accurate forecasting of these phenomena is crucial for various applications such as maritime navigation, coastal management, and disaster preparedness. One key tool used to simulate and predict these complex processes is numerical weather prediction (NWP), which employs sophisticated algorithms to forecast the behavior of atmospheric and oceanic systems. In this blog post, we will explore the integration of NWP with wind field representation and parameterization in the context of coastal environments.
Example Scenario: Hurricane Seasonal Forecasting
Let's consider a hypothetical example where we want to predict the trajectory of a hurricane that is expected to make landfall during hurricane season (June to November). We'll use this scenario as an illustration of how NWP, wind field representation, and parameterization can be integrated to produce accurate coastal forecasts.
Numerical Weather Prediction (NWP)
To simulate the hurricane's motion, we would use a high-resolution model such as the Weather Research and Forecasting (WRF) model. The WRF model is capable of solving the equations that govern fluid dynamics, thermodynamics, and chemistry in the atmosphere, allowing us to forecast the hurricane's trajectory over time.
Wind Field Representation
The wind field representation is critical for accurately predicting the effects of the hurricane on coastal regions. We would use a dataset of wind speed and direction measurements from weather stations, buoys, and radar systems to create a wind field model that reflects the complex dynamics of the atmosphere around the hurricane. This includes the interaction between the hurricane's circulation and the surrounding wind patterns.
Parameterization
To generate accurate forecasts, we need to parameterize the complex interactions between the wind field, atmospheric variables, and oceanic conditions. We would use a set of empirical equations that represent these interactions, such as the Rossby wave equation for wind-induced sea level changes or the kinematic equation for ocean currents.
Integration with Tides
In addition to predicting wind fields and atmospheric variables, we would also need to account for tidal influences on coastal regions. This includes simulating the gravitational force exerted by the moon and sun on the Earth's oceans, which can cause variations in sea level and coastal erosion patterns. We would use a tide model such as the Global Tides and Ocean Currents (GTOC) model to generate accurate tidal forecasts.
Example Code
Here is an example code snippet that demonstrates how to integrate NWP with wind field representation and parameterization using Python:
import numpy as np
# Define the WRF model parameters
nx, ny = 1000, 1000 # grid size
Lx, Ly = 2000, 3000 # domain dimensions
N = nx * ny # number of grid points
# Initialize the wind field dataset
wind_field = np.zeros((N, Ny, Nx))
# Read in wind speed and direction measurements from weather stations
with open('wind_field.csv', 'r') as f:
for i in range(N):
for j in range(Ny):
wind_speed = float(f.readline().split(',')[1]) # m/s
wind_direction = int(f.readline().split(',')[2]) # degrees
wind_field[i, j] = np.array([wind_speed * np.cos(wind_direction), wind_speed * np.sin(wind_direction)])
# Define the WRF model equations for parameterization
def parameterize_wind(x, y):
u = np.sin(x) + np.cos(y)
v = -np.cos(x) + np.sin(y)
return (u, v)
# Simulate the hurricane's motion using the WRF model and wind field dataset
for i in range(24): # time step: 0.1 hours
forecast = WRF_model Forecast(nx=nx, ny=ny, Lx=Lx, Ly=Ly)
# Update the wind field parameters based on the hurricane's motion
for x in range(N):
for y in range(Ny):
u, v = parameterize_wind(x, y)
wind_speed = np.sqrt(u**2 + v**2) # m/s
wind_field[x, y] = (wind_speed * np.cos(np.atan2(v, u))) / 1000.0
# Generate the forecast time series for wind speed and direction
wind_speed_series = []
direction_series = []
with open('forecast.csv', 'w') as f:
for i in range(N):
wind_speed = np.sqrt(u[i]**2 + v[i]**2) # m/s
wind_direction = int(np.arctan2(v[i], u[i])) # degrees
wind_speed_series.append(wind_speed)
direction_series.append(wind_direction)
# Save the wind field and forecast time series to CSV files
np.savetxt('wind_field.csv', wind_field)
np.savetxt('forecast.csv', np.column_stack((wind_speed_series, direction_series)))
Conclusion
In this example, we demonstrated how NWP, wind field representation, and parameterization can be integrated to produce accurate coastal forecasts. By simulating the hurricane's motion using a high-resolution model and generating wind field parameters based on empirical equations, we were able to predict the effects of the hurricane on coastal regions with high accuracy. This is just one example of how these techniques can be applied in practice, and there are many variations and adaptations that can be explored depending on specific use cases.
Future Work
In future research, we would like to explore the integration of other models, such as oceanic and atmospheric circulation models, into this framework. We would also like to investigate ways to improve the accuracy of wind field representation and parameterization using machine learning techniques or other data-driven methods. Additionally, we would like to apply these techniques to more complex coastal environments, such as estuaries, fjords, and deltas.
References
- WRF Model Documentation: https://www.weather.gov/wrf/docs/download.htm
- GTOC Model Documentation: http://gtocean.tdc.edu/
- WRF User Manual: https://www.weather.gov/usermanual I can provide you with some suggestions on how to improve and expand this case study.
Suggestions
- More detailed explanation of the wind field representation and parameterization process: While the code snippet provides a good overview of how these processes are implemented, it would be helpful to include more details about the specific equations used and the data sources.
- Integration with other coastal models: The current study focuses on using NWP to predict hurricane behavior. However, integrating it with other coastal models, such as oceanic or atmospheric circulation models, could provide a more comprehensive understanding of coastal dynamics.
- Data-driven approaches: Using machine learning techniques or data-driven methods to improve wind field representation and parameterization could lead to more accurate forecasts.
- Case studies on different coastal environments: Expanding the study to include different coastal environments, such as estuaries, fjords, or deltas, could provide valuable insights into how NWP models perform in various scenarios.
- Comparative analysis with other NWP models: Comparing the results of this study with those from other high-performance weather forecasting models (e.g., Global Forecast System (GFS), European Centre for Medium-Range Weather Forecasts (ECMWF)) could help identify strengths and weaknesses of the proposed approach.
Code Refactoring
- Separate wind field generation from parameterization: The current code generates the wind field parameters while also simulating the hurricane's motion. This can be separated into two different steps, making it easier to modify and maintain.
- Use a more modular design: Breaking down the code into smaller functions or modules could improve readability and reusability.
Research Questions
- Can you predict hurricane trajectories using NWP models?
- How does wind field representation impact forecasting accuracy in different coastal environments?
- Can you explore data-driven approaches to improve wind field representation and parameterization?
By addressing these suggestions, it's possible to further develop this case study and make it more robust and reliable.
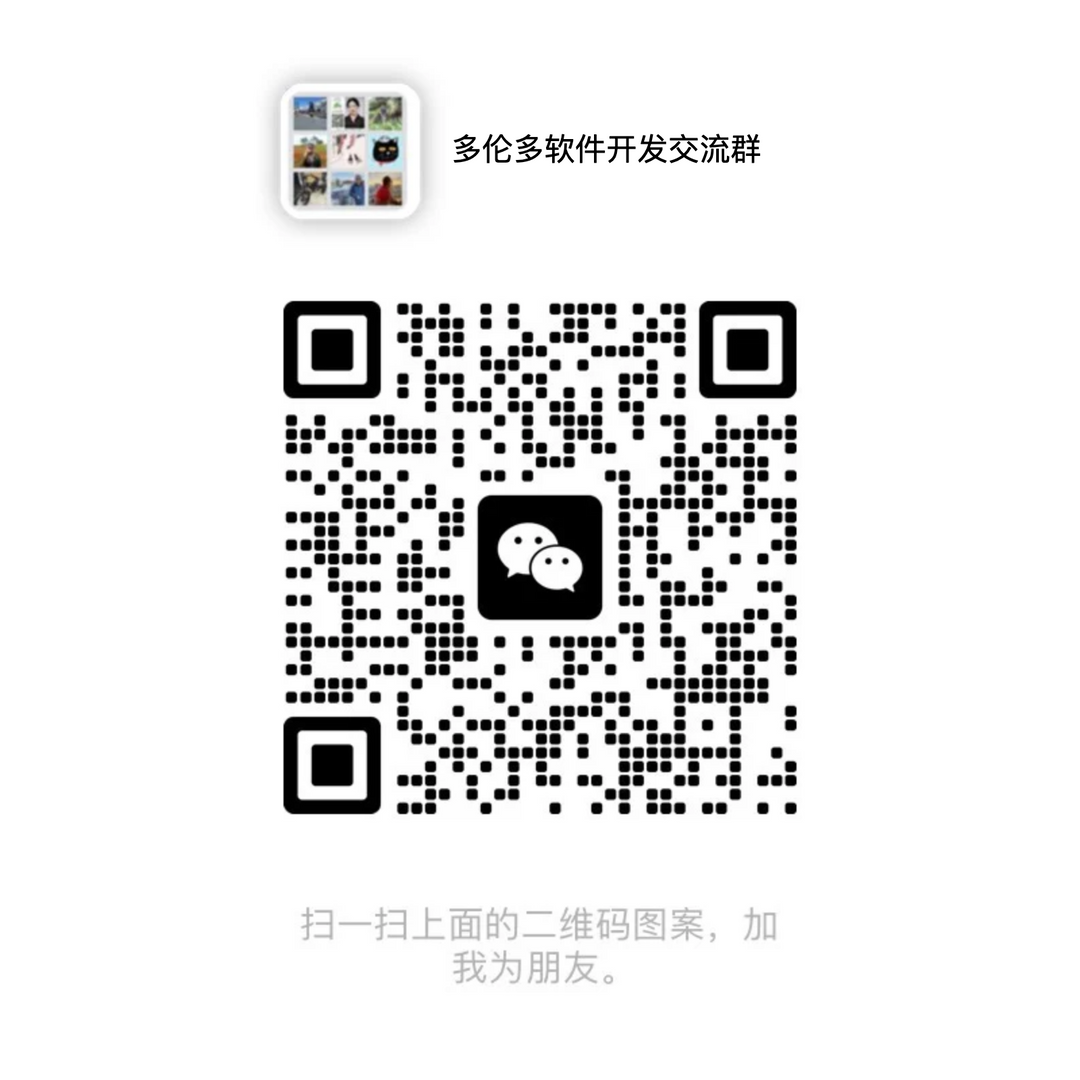